ASP.NET Web Forms extensively use postback mechanism in order to maintain the state of the server-side controls on the web page. This makes it somewhat tricky to perform URL rewriting for ASP.NET pages. When a server side form control is added to the web page, ASP.NET will render the response with HTML <form> tag that contains an action attribute pointing back to the page where the form control is. This means that if URL rewriting was used for that page, the action attribute will point back to the rewritten URL, not to the URL that was requested from the browser. This will cause the browser to show rewritten URL any time a postback occurs.
Let me demonstrate this on an example. Assume you have a very simple web form in a file called default.aspx. When you request http://localhost/default.aspx in a browser and then view the HTML source for the response, you will see that the response contains the <form> element, which looks similar to this:
<form name="form1" method="post" action="Default.aspx" id="form1">
The action attribute contains the URL where the form data will be posted to when you click on the button in the web page.
Now, let’s create a very simple rewrite rule that rewrites URL from http://localhost/homepage to http://localhost/default.aspx.
<rewrite>
<rules>
<rule name="MyRule" patternSyntax="Wildcard">
<match url="homepage" />
<action type="Rewrite" url="default.aspx" />
</rule>
</rules>
</rewrite>
When you request http://localhost/homepage in a browser, the URL will be rewritten in accordance to the above rule and the page will be shown correctly in the browser:
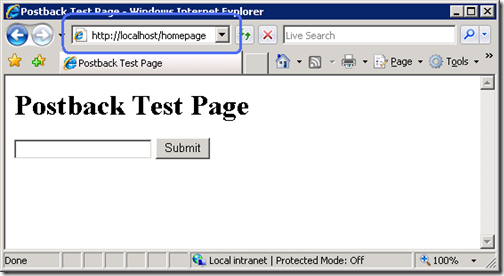
However, when you click on the Submit button, the browser’s address bar will display http://localhost/default.aspx, thus exposing the internal URL, that you wanted to hide by using URL rewriting:
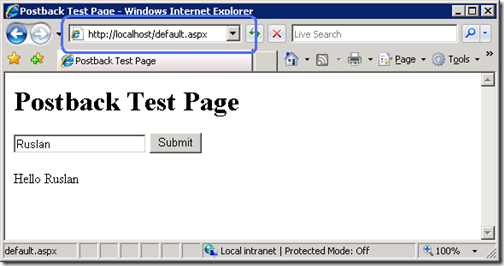
A few workarounds to fix this behavior existed in previous versions of ASP.NET. For example, you could sub-class the form control, or use Control Adapter as explained here. However, these workarounds were not very easy to implement. Luckily, the ASP.NET in .NET Framework 3.5 SP1 provides a very simple way to fix that. Now you can use the property of the HtmlForm class called Action to set the postback URL to the one that was requested by browser before any rewriting happened. In ASP.NET you can obtain that URL by using HttpRequest.RawUrl property. So, to fix the postback URL for your web form when you use URL Rewrite Module, you would need to add the following code to the page:
protected void Page_Load(object sender, EventArgs e)
{
form1.Action = Request.RawUrl;
}
After this change, if you reload the web page at http://localhost/homepage and then click on submit button you will see that the browser’s address bar still displays the correct URL:
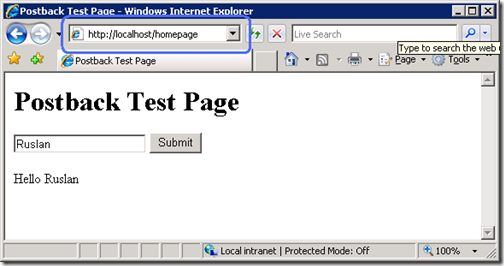
If you view the HTML for the response you will see that the <form> tag now contains the correct action URL:
<form name="form1" method="post" action="/homepage" id="form1">
When you use ASP.NET master pages you could add the Page_Load method to the master page and that would take care of postback action URL for all the pages in your web application. Note thought that in order to be able to use the HtmlForm.Action property you have to upgrade to .NET Framework 3.5 SP1.
Wasted 2 hours before I found this solution. I can’t understand that this is not mentioned under “Using the module” in the http://learn.iis.net/page.aspx/460/using-url-rewrite-module/ page. I would guess that I am not the only one looking for this solution.
Thanks for the blog!
MadMan, thanks for feedback! I will make sure the articles at learn.iis.net will have this information.
respected sir,
I am new to ASP.NET. I am working on URL routing for last couple of weeks, although i have implemented routing on the static pages in my site, but i get stuck when i have to implement it on dynamic URL’s.
eg
If i have to generate url’s for the each user profile in my site, then the relative URL which needs to be generated should not contain the query string values such as the user id.
secondly
if i have two pages( home.aspx and profile.aspx) in my site in the same folder eg “logged”. The actual Url is mysite/logged/home.aspx.
i want the relative url to be mysite/index, which i have achieved using your code but now when i click on some other page and then when again i hit in the home tab the url displayed is mysite/logged/index which is wrong. The folders name is shown again in the url.
what should i do? hope you understand what i want to achieve.
regards
prabhjot
prabhjot,
Does your ASP.NET application use “~” symbols when constructing the URLs for the home tab? If yes, then this may be due to a bug in ASP.NET that we will fix by the time we ship RTW of the URL rewrite module.
More information about URL rewriting for ASP.NET web forms is available here: http://learn.iis.net/page.aspx/517/url-rewriting-for-aspnet-web-forms/
Awsom man…
Thanks a lot..I was lucky enough not to waste time that others have wasted.
Nilesh Patel.
Thanks a lot for this article.
I am working with .NET 2.0 and tried using the code as you have posted. It worked just fine.
Now I am wondering why you mentioned that it only works with .NET 3.5 SP1?? Is there something I am missing here?
As far as I know the Action property of the HtmlForm class did not exist until .NET Framework 3.5 SP1. If you are able to use this property then you must be using 3.5 SP1.
If you can’t update your Framework, you still can change tha form action using javascript.
Just put the HttpRequest.RawUrl value in any hidden input and then change de form action with something like this (using jquery because of the possibility to find tags by class):
$(document).ready(function(){
$(“.masterForm”).attr(“action”, $(“.rawUrl”).val());
});
not ellegant, but works.
Thanks so much – I had tried for days to get https redirection to work – the documentation is just vague enough to get me close but no results – your example worked the first try!
Thanks, that fixed my problem..
@Ruslany: FYI, I’m targeting .Net 2.0 and HtmlForm.Action property was present.
Thanks a lot! it was a great help………..
In our web page we use the following to remove default.aspx.
We use as above we get post action=”/default.aspx” and the post back is not working for the home page.
so we made the following change.
form1.Action = Request.RawUrl.Replace(“default.aspx”, “”)
so we made the modification,
We just moved our development boxes from IIS6 to IIS7 and were eager to replace our home grown url rewrite with IIS7 rewrite. .Net 3.5 SP1 is installed. The rewrite is working as expected, no issues, but we are unable to retrieve the original url and update the postback url. Both Request.RawUrl and Request.ServerVariables(“HTTP_X_ORIGINAL_URL”) contain the rewritten URL instead of the orginal URL. What did we miss?
TIA,
Bill
Bill, did you install .net 3.5 SP1 after URL rewrite? If yes, then you will need to re-install the URL Rewrite so that it applies the patch for the ASP.NET which fixes the Request.RawUrl behavior.
i have integrated url rewriting with 1.1 module with iis 7
Its working fine for static pages.
But i have some dynamic pages where page is depend on querystring.
i have to pass dynamic querysting from web.config in rule that i have defind
how should i achieved this.
any idea ?
@Jitendra: please send me the examples of input URL and what it should be rewritten to. You can use the contact form on this blog.
Hi ruslany,
I have Using URL Rewriting.Net Dll in my Project.
I have completed all Rewriting but I have One Issue, that is Postback Button click is not happaning in my Project after applying Rewriting rule.
I am Not able to set “form.Action = request.rawURL” because all the Pages is loading Dynamic and on the base of Querystring.
Example : – “MY Current Url: http://www.mydomain.com/home.aspx?pid=46”
I want to Rewriting as a http://www.mydomain.com/home.aspx/Calender or http://www.mydomain.com/home.aspx/Calender.aspx.
please provide me a proper solution for Button Click Event.
waiting for your reply
Regards
Hemant Tawri
@Hemant: I am not that familiar with URL Rewriting.NET. Have you tried using the IIS URL Rewrite Module instead? URL Rewrite 2.0 has a feature for changing the URL’s in the response HTML. You could use it to change the form.Action element in the response.
Hi Ruslany,
We are mixing a lot of Asp.Net technologies in a large web environment. In approximately 6 months we will be moving over to IIS 7.5 (and Url Rewrite 2.0 off course), but for the moment we are still using IIS 6…and trying to incorporate a rewriting solution.
The issues is that we came accross the famous “~ operator in ASP.NET Web server control is resolved incorrectly ” bug. You mentioned the Asp.Net kind-of-hotfix that takes place underneath the installation of Url Rewrite. A few questions:
– Is this hotfix available for IIS 6 as well?
– If not, is it possible to work around the issue? (keeping in mind that the usage of ResolveClientUrl and alike is widespread in the framework. And that we have a large codebase.)
– Are there other strategies/alternatives (on IIS 6)
Also note that the we are familiar with the various articles on the net (like scott mitchells old one, adapter strategies and the bug listed on connect with id 235385). In particular we fear sudden changed postback actions and alike…
Regards and keep up the good work,
WP
Hi WP:
The hotfix for ASP.NET that is included with URL Rewrite is not applicable to IIS 6.
I cannot think of a good workaround for that. One option may be to not use ~ operator and instead rely on absolute URL paths or to sue HTML <base> tag? But it will require substantial changes to the application code, which I am not sure you can afford.
Hi ! I implemented your way and it works for certain postbacs but not for login button. When I press the login buttun it has gotten the ugly url again;
login.aspx?returnurl=/transfers/transfer-description.aspx%3ftransfertype%3d6
what should I do here??
Hi tugberk_ugurlu, which .NET version and OS do you have? If I remember correctly the login control behavior related to URL Rewriting was fixed in Windows 7 (Windows Server 2008 R2).
Brilliant!
Many thanks!
great, thanks very much
WOOOWWW!!!! Thanks, man! I’ve been looking for this for the whole morning!!!
Hello sir,
I want to some help to you if you dont mind,
i m making a website like this site http://bit.ly/,using asp.net and sql server 2005,
and my server is IIS 7 , and i m already install helicon ape which provide url rewriting.
but its not working, can help me how can do this. Please expalin and gives the details step by step.
Please send details on my mail id .
Thanks in advance
savi
Very very much thanks man
Check this link
http://www.mindstick.com/Articles/9992a0bc-90f5-4f04-823a-31f901b61643/?URL Routing in ASP Net 3 5 IIS7
I have a bad time in rerouting non www version to www version. Finally i used a 301 permanent redirect in code behind.
Hemant,
I suppose you are using Intelligencia dll
Use this register tag in your master pages:
[XML fragment has been removed because it was not XML encoded]
and rename your tag to
[XML fragment has been removed because it was not XML encoded]
now you should be able to do the postbacks
Cheers,
Sam.
Hello Ruslany,
In your March 2, 2010 posting you state: “URL Rewrite 2.0 has a feature for changing the URL’s in the response HTML. You could use it to change the form.Action element in the response.”
I am redirecting /default.aspx to the domain root and my controls are not working due to the postback issue. I placed the Form.Action = Request.RawUrl in the Page Load method of the default.aspx code behind but no success.
Can you please tell me, or point me to an article that will show me, how to change the form.Action element using URL Rewrite 2.0 in IIS7?
Thanks for all the great work that you do.
Louis in Chicago
Hi Louis. This article should give you an ideal how to do this. It shows how to rewrite the link URL, but you can use the same approach to rewrite the url in the action attribute of the form tag.
Thanks for the quick reply Ruslany. I was actually able to correct the problem by adding the following statement in the default.aspx code behind file:
Form.Action = Request.RawUrl.Replace(“default.aspx”, “”)
I’m not sure why the original Request.RawUrl statement did not work. All is working properly now.
Best regards,
Louis
Hello Ruslany,
I’m using URLRewrite module of IIS 7
All my pages are rewritten from /
to /htmls/.aspx.
My Website default document is therefore: htmls/home.aspx
When I submit a standard (without runat=server tag) form in the homepage, form fields are not sent back to the Homepage.
ASP.Net form (with runat=server tag) fields are posted-back well though.
What might be the reason for that?
Thanks in advance
Yossi (Israel)
I added tags in the previous message that probably caused some content removal, here is the corrected section (without the tags):
******
All my pages are rewritten from MyDomain/MyPage
to MyDomain/htmls/MyPage.aspx
My Website default document (as specified in IIS manager) is therefore: MyDomain/htmls/home.aspx
******
Yossi
Hi Yossi, the form tag has to have the runat=”server”. This causes ASP.NET to process the form element during generation of HTTP response and to replace the URL in the action attribute. Without this ASP.NET will not touch the form element.
I has a doubt……..I want to rewrieUrl with Images….\
Actually we have done this concept but Images r not displaying….all are fine except Images and JQuery Concepts
data is coming but images ae not coming
Could any one please suggest about this problem.
Thanks !!!!!!!!!!!…………..
Hello,
I am new to IIS 7.5 I have done the URL Rewirte Module 2.0 in my asp.net application.
but i have to still go forward learn
because, when i try to use http://technofast.ganees.com/ganees to redirect to mypage.aspx?ownpagename=ganees
how do i write the rule to match url here “ganees” is dynamic name that need to access via request.querystring[“ganees”].toString() in code behind
please help to observe this concept.
Thanks in Advance
with regards,
prabhakaran
I have an issue in my asp.net web site. Sometimes button click events are not firing and sometimes it is firing.
You can check in the live site also. Here is the link http://www.enjoysharepoint.com/sendmail.aspx .Here if you can send mail to fewlines4biju@gmail.com with ant text. If the events will properly fire then it will not clear the text, but it will show a message “Mail Sent !!!” below to send mail button.
But if the event will not fire then it simply clear the text boxes.
What I feel is that, after I have implemented URL rewritting by using Intelligencia.UrlRewriter.dll , the behaviour changes to this.
Please let me know any solution for this.
If you will try for 2/3 times, the event is firing.
Waiting for ur replies!!!
Third time that you have saved me hours worth of troubleshooting! The code “form1.Action = Request.RawUrl;” in my codebehind page fixed the issue as you described.
Thanks again!!!
Thanks. The whole article was something I’ve done it before, many times. But, I didn’t know about Request.RawUrl. What I’ve done in my previous projects, was to create a custom form control, and set the action attribute of that, in overridden Render method.
However, this method is really nice and clean. Thanks.
Hi Ruslany,
Is this behavior fixed in Microsoft URL Rewrite Module 2.0 for IIS 7 (x64) ? Do we have to still use the fix “form.Action = Request.RawUrl;” ?
Please let us know.
Thanks, Karthik
Can you attach the code used in this article please
Thanks a lot for the great article, Ruslany. Its very simple and easy solution. It saved my lots of time….
Thanks again!!
Thanks a lot for valuable info….:)
thank a lot!!!!!!!!!!
Mr. Ruslany, I’ve been using info from your blog in my ASP.NET MVC 3 app…in particular some of the rewrite rules from your ’10 URL Rewriting Tips and Tricks’ post, and you’ve been an enormous help. However, I’ve run into a problem with using the POST method in forms. It seems that using the rule to rewrite ‘http’ to ‘https’ is somehow changing the request from a POST to a GET, and then I get a ‘404 Not Found’ error. Does this sound familiar to you? Is there any fix for this? I have posted a question on StackOverflow for this problem, if you’d be inclined to take a look. Thanks!
while using your code i am getting the below error
Validation of viewstate MAC failed. If this application is hosted by a Web Farm or cluster, ensure that configuration specifies the same validationKey and validation algorithm. AutoGenerate cannot be used in a cluster.
Actually what i want to do..
When a member(suppose neetu) login on a page s/he will be redirected on index.html page with the url
http://www.mysite.com/member/neetu
neetu(id) is coming from database and member is a folder i created
so i m trying this with url rewriting and routing too…..but nothing works…can u plz help me…its urgent and i am using asp.net 2.0/visual studio 2005 without ajax
Hi
thanks for the article.
I need to redirect to a URL but avoid all the images present on the URL.
Is it possible to do in C#.NET ?
Also, is there any tool present to perform testing of URL redirection in local development environment?
Thanks..
Hello Sir,
I have developed a dynamic website with some static page. I have used most of the bind controls like listview.I have a lot folder hierarchy, please provide me a url rewriting solution immediately. Its very urgent.
Thanks in advance.
thank you SO MUCH!!!
Hi! Mr. Ruslany. I am Sukhdev Singh.
Greetings of the Day.
I want to know if i have 50 Pages with unique Page Name for a website, then shall i need to rewrite URL script for each page in config file. i don’t think so.
Is there any default rule or module settings in IIS which is applicable on each page of various web sites and there linked pages.
Regards:) Sukhdev Singh
Great post, thank you very much! Just faced that problem and were lucky enough to come across your post 🙂 Excellent job!
Hi! Mr. Ruslany
My case looks to be a bit different, but sure that you can address it. I want to offer affiliates to market my site. However when affiliate redirects the visitor to my site, i want to create an illusion as if the visitor is on affiliate’s site with urls rewritten with affilliates home url + something.
how to hide url at the bottom of the page in asp.net when use the postbackurl for linkbuutton when i mouse over on linkbutton
onmouseover=”window.status=”;”
I have been trying to do a 301 Permanent Redirect instead of Rewrite through URL Rewrite for removing “default.aspx” from the URL for SEO purposes. However, when the page posts back, the URL Rewrite Rule kicks in, redirects back to default.aspx and stops processing, thereby not letting the postback code for the button execute. I tried replacing “default.aspx” with “” in RawURL and setting that as Form.Action in Page_Load. But, the URL Rewrite rule kicks in even before the Page_Load code can be executed. Any idea on how to resolve this?
worked !! thank you so much
Dear Sir,
i wasted 2 hours before i found this solution.
Thanx a lot sir .
sir i also face a problem when we use url rewrite module on below url
Current Url:
https://epay.apollohospdelhi.com/DisplayProduct.aspx?id=7
Required Url:
https://epay.apollohospdelhi.com/health-checks/Child-Health-Check.aspx
then all path are change because of “health-checks” directory , please mail me solution .
Thanx & Regards
Anurag Soni
Good!
Hi Ruslany,
My question is related to url redirect. When user enters the https://www.subdomain.domain.com then it redirect to https://subdomain.domain.com but its showing privacy error and i had also configured in the web.config file but still its not redirecting and had taken the wildcard ssl certificate for my domain.
in cofig file:
<!—->
<!—->
Thanks for the post, I wanted to redirect from a query string parameter to particular product name.
I fixed by following your URL. Very useful post, Thanks Rusian for the post.